Here we will show how to use our Rotating Proxy with Selenium Chrome/Firefox. You can use both our Rotating Premium Proxy services (stable premium proxies) and Rotating Open Proxy (unstable public proxies) for Selenium browsers.
Proxy Authentication
Selenium doesn’t support username:password
authentication of a proxy natively. Some plugins may help you, such as this one (for node.js) or this one (for Python). Here we will use IP authentication without any plugin.
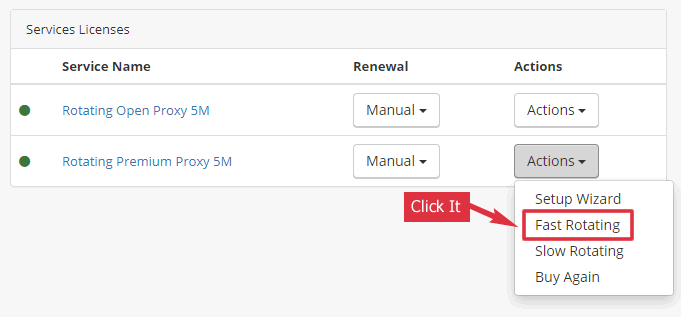
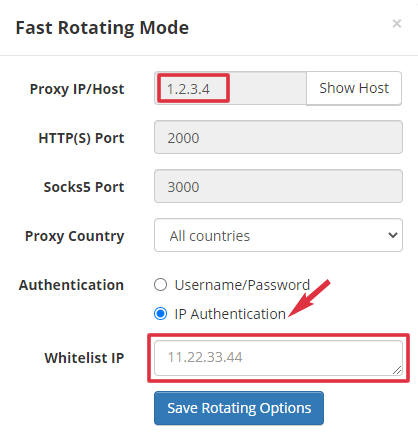
Selenium Chrome Proxy
Here are some codes showing how to use our rotating proxy with Selenium Chrome. In the code, we use 1.2.3.4
as the demo proxy IP. You should use the real one got from the client area.
We use the URL http://www.find-ip.net and http://checkip.amazonaws.com for the test. They show their visitor’s IP. You should see a new IP every time you use our rotating proxy to access it.
import org.openqa.selenium.Proxy; import org.openqa.selenium.WebDriver; import org.openqa.selenium.chrome.ChromeDriver; import org.openqa.selenium.chrome.ChromeOptions; public class proxyTest { public static void main(String[] args) { Proxy proxy = new Proxy(); proxy.setHttpProxy("1.2.3.4:2000"); ChromeOptions options = new ChromeOptions(); options.setCapability("proxy", proxy); WebDriver driver = new ChromeDriver(options); driver.get("http://www.find-ip.net"); driver.manage().window().maximize(); driver.quit(); } }
from selenium import webdriver PROXY = "1.2.3.4:2000" # demo proxy chrome_options = webdriver.ChromeOptions() chrome_options.add_argument('--proxy-server=%s' % PROXY) chrome = webdriver.Chrome(options=chrome_options) chrome.get("http://checkip.amazonaws.com") body_text = chrome.find_element_by_tag_name('body').text print(body_text) chrome.quit()
using OpenQA.Selenium; using OpenQA.Selenium.Chrome; public class ProxyTest{ public static void Main() { ChromeOptions options = new ChromeOptions(); Proxy proxy = new Proxy(); proxy.Kind = ProxyKind.Manual; proxy.IsAutoDetect = false; proxy.HttpProxy = "1.2.3.4:2000"; proxy.SslProxy = "1.2.3.4:2000"; options.Proxy = proxy; options.AddArgument("ignore-certificate-errors"); IWebDriver driver = new ChromeDriver(options); driver.Navigate().GoToUrl("https://www.find-ip.net"); driver.Quit(); } }
require 'rubygems' require 'selenium-webdriver' proxy = Selenium::WebDriver::Proxy.new(:http => "1.2.3.4:2000") driver = Selenium::WebDriver.for :chrome, :proxy => proxy driver.navigate.to "http://www.find-ip.net" driver.quit
let webdriver = require('selenium-webdriver'); let chrome = require('selenium-webdriver/chrome'); let proxy = require('selenium-webdriver/proxy'); let opts = new chrome.Options(); (async function example() { opts.setProxy(proxy.manual({http: '1.2.3.4:2000'})); let driver = new webdriver.Builder() .forBrowser('chrome') .setChromeOptions(opts) .build(); try { await driver.get("http://www.find-ip.net"); } finally { await driver.quit(); } }());
import org.openqa.selenium.Proxy import org.openqa.selenium.WebDriver import org.openqa.selenium.chrome.ChromeDriver import org.openqa.selenium.chrome.ChromeOptions class proxyTest { fun main() { val proxy = Proxy() proxy.setHttpProxy("1.2.3.4:2000") val options = ChromeOptions() options.setCapability("proxy", proxy) val driver: WebDriver = ChromeDriver(options) driver["http://www.find-ip.net/"] driver.manage().window().maximize() driver.quit() } }
Selenium Firefox Proxy
Here are some codes showing how to use our rotating proxy with Selenium Firefox. In the code, we use 1.2.3.4
as the demo proxy IP. You should use the real one got from the client area.
import org.openqa.selenium.Proxy; import org.openqa.selenium.WebDriver; import org.openqa.selenium.firefox.FirefoxDriver; import org.openqa.selenium.firefox.FirefoxOptions; public class proxyTest { public static void main(String[] args) { Proxy proxy = new Proxy(); proxy.setHttpProxy("1.2.3.4:2000"); ChromeOptions options = new FirefoxOptions(); options.setCapability("proxy", proxy); WebDriver driver = new FirefoxDriver(options); driver.get("http://www.find-ip.net"); driver.manage().window().maximize(); driver.quit(); } }
from selenium import webdriver firefox_capabilities = webdriver.DesiredCapabilities.FIREFOX firefox_capabilities['marionette'] = True proxy = "1.2.3.4:2000" firefox_capabilities['proxy'] = { "proxyType": "MANUAL", "httpProxy": proxy, "ftpProxy": proxy, "sslProxy": proxy } driver = webdriver.Firefox(capabilities=firefox_capabilities) driver.get("http://checkip.amazonaws.com") body_text = driver.find_element_by_tag_name('body').text print(body_text) driver.quit()
using OpenQA.Selenium; using OpenQA.Selenium.Firefox; public class ProxyTest{ public static void Main() { FirefoxProfile profile = new FirefoxProfile(); Proxy proxy = new Proxy(); proxy.Kind = ProxyKind.Manual; proxy.IsAutoDetect = false; proxy.HttpProxy = "1.2.3.4:2000"; proxy.SslProxy = "1.2.3.4:2000"; options.Proxy = proxy; profile.SetProxyPreferences(proxy); FirefoxDriver driver = new FirefoxDriver(profile); driver.Navigate().GoToUrl("https://www.find-ip.net"); driver.Quit(); } }
require 'rubygems' require 'selenium-webdriver' include Selenium profile = WebDriver::Firefox::Profile.new profile["network.proxy.type"] = 1 profile["network.proxy.http"] = "1.2.3.4" profile["network.proxy.http_port"] = 2000 driver = WebDriver.for(:firefox, :profile => profile) driver.navigate.to "http://www.find-ip.net" driver.quit
var webdriver = require('selenium-webdriver'), proxy = require('selenium-webdriver/proxy'); var driver = new webdriver.Builder() .withCapabilities(webdriver.Capabilities.firefox()) .setProxy(proxy.manual({http: '1.2.3.4:2000'})) .build(); driver.get('http://www.find-ip.net');
import org.openqa.selenium.Proxy import org.openqa.selenium.WebDriver import org.openqa.selenium.chrome.FirefoxDriver import org.openqa.selenium.chrome.FirefoxOptions class proxyTest { fun main() { val proxy = Proxy() proxy.setHttpProxy("1.2.3.4:2000") val options = FirefoxOptions() options.setCapability("proxy", proxy) val driver: WebDriver = FirefoxDriver(options) driver["http://www.find-ip.net/"] driver.manage().window().maximize() driver.quit() } }